[教學]常用處理字串的方法
整理系列 - String
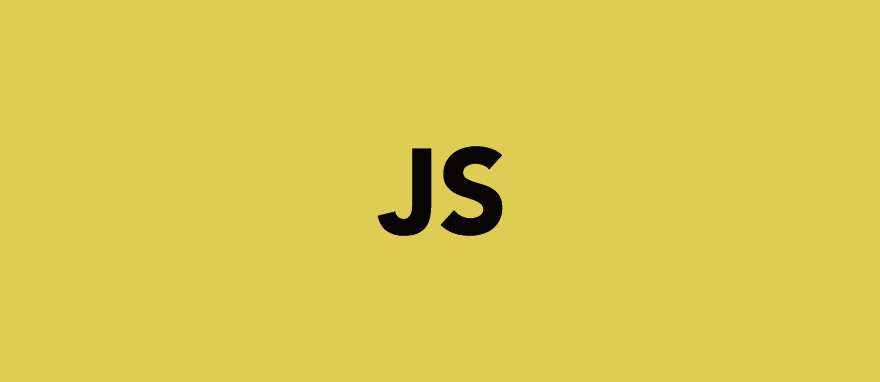
前言
每次都會忘記字串、物件、陣列的方法該怎麼使用,於是我決定花些時間記錄下來,以下是常用處理字串的方法。
目錄:
合併:concat()
concat()
方法將一個或多個字串與原字串連接合併,形成一個新的字符串並回傳。意思等同用加號相加,MDN文件強烈建議使用「賦值運算子」也就是(+
, +=
)來代替此方法。
分割:split()
split()
方法使用指定的分隔字,將字串分割成子字串,並以陣列的方式回傳。
分割:substring()
substring()
方法會回傳指定位置之間的字串。
我們可以運用字串長度的性質來做處理,像是「列出字串的後5位數」等。使用字串長度顯然更輕鬆,因為不需要去記住起始值與最終值,只需扣掉要的長度即可:
分割:slice()
slice()
方法提取部分字串並以字串的方式回傳。概念像從某個位置切下去。
分割:substr()
substr()
方法會回傳一個由指定位置開始算長度的字串。與上方兩者不太相同,擷取字串並非設定以索引值設定頭尾來取,反之,以頭的索引值開始,擷取內容以長度來區分。
找值:indexOf()
indexOf()
方法與其說找值,不如說它能告訴你想要找的值存不存在。存在會回傳該值的第一個索引值;若不存在就會回傳-1,
找值:lastIndexOf()
lastIndexOf()
方法與 indexOf()
方法相似,差別只在於 lastIndexOf()
只會回傳鎖定對象最後一次出現的索引值。
找值:search()
search()
方法與 indexOf()
方法相似,差別在於 search()
方法可以使用正規表達式。
找值:includes()
includes()
方法不能全然說他能找值,只能說它能判斷你要找的值是否有出現,因為它的回傳值是布林。
取代:replace()
replace()
方法能取代特定文字。
去除空白:trim()
trim()
方法能夠清除字串兩端的空格(包含tab)。
轉大寫:toUpperCase()
toUpperCase()
方法能將字串全部轉為大寫,若非字串是會強制轉型(null
、undefined
會報錯 )。
轉小寫:toLowerCase()
toLowerCase()
方法能將字串全部轉為小寫,若非字串是會強制轉型(null
、undefined
會報錯 )。
結語
以上整理了常用的字串方法,接下來幾篇應該是整理陣列、物件的方法。